Step 3: Sending Assets
Whitelisted RecipientIf the recipient of your transaction has not followed Step 1 - Whitelist your Vault, then all transactions being sent to their address will fail.
1. Initiating a Transfer
To create a transaction request moving assets on the io.network use the /v1/vaults/{vaultId}/network/transfer
endpoint. Here the contract address refers to the asset that you are trying to send, and is a static value that will never change. To obtain the contract address you can use the /v1/vaults/{vaultId}/assets
endpoint or contact support.
const response = await fetch(`https://api.iofinnet.com/v1/vaults/${vaultId}/transactions`, {
method: 'POST',
headers: {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json'
},
body: JSON.stringify({
contractAddress: '0xd386b09A7083BAA1986A25206A7F59CcC6DC8C76',
amount: '100.01',
recipientAddress: '0xd88F29AF6C3F87f1B98931Df4079Cda85A7Fe125'
})
});
const resJson = await response.json();
Public BlockchainsIf you are attempting to send assets on a public blockchain such as Bitcoin or Ethereum, use
/v1/vaults/{vaultId}/{chainAlias}/transfer/
instead.
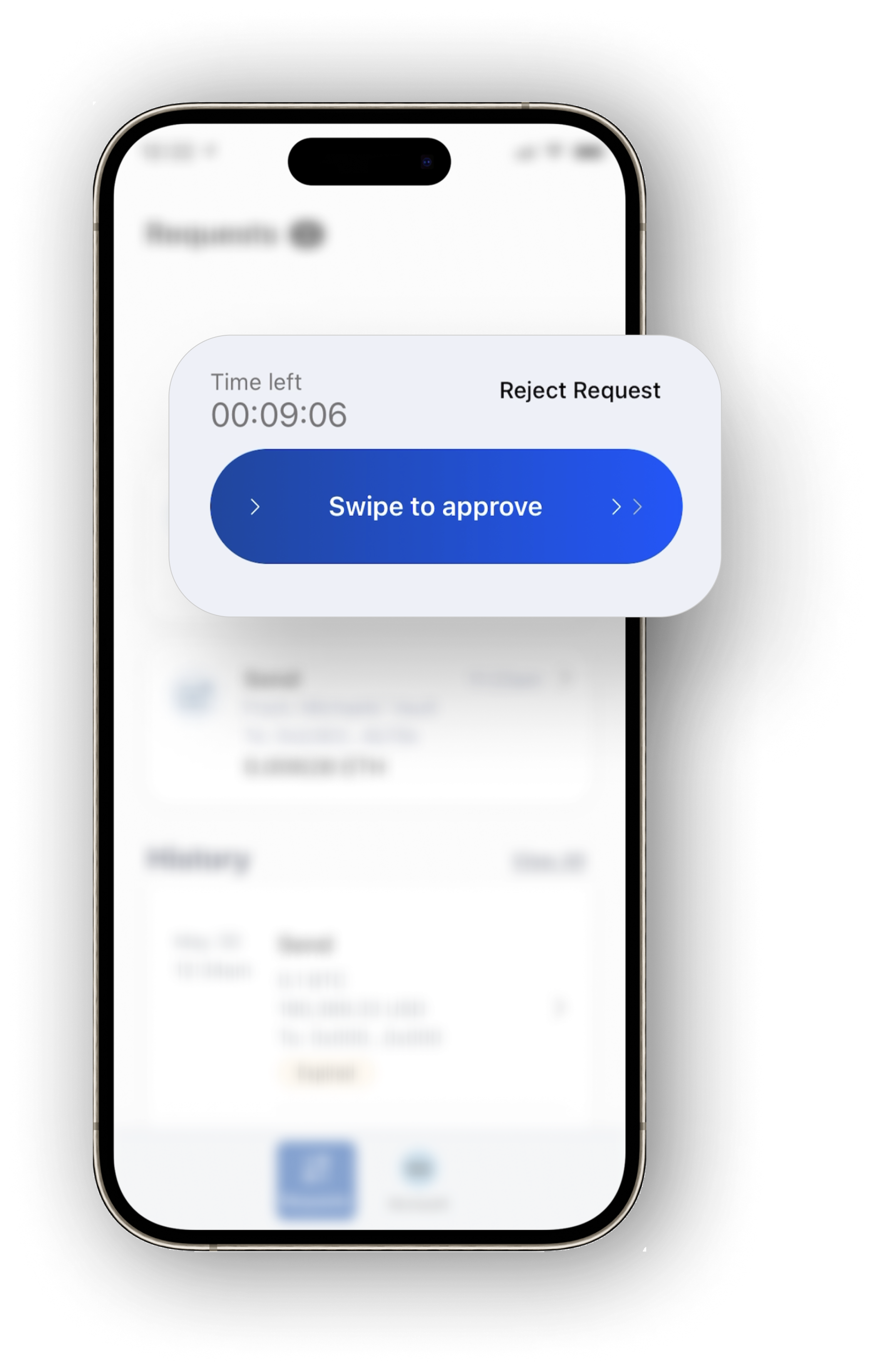
MPC Approval
Vaults are secured by our unique trustless MPC cryptography. To complete a transaction, the signers of the vault need to approve the request. Once approved, their devices will participate in the 'signing' ceremony, and upon completion produce a fully signed transaction. This means that our API's create 'requests' which still follow the signer approval flow.
Now that we've hit the endpoint and created the transfer request, all of the signers on the vault should receive a request for approval. Once approved, the signed transaction will broadcast and become final within seconds.
To automate this flow follow step 4 to see how a virtual signer can be utilised.
2. Tracking the Finality (TODO)
Once the transaction is submitted, you can track its status using the /v1/vaults/{vaultId}/transactions/{transactionId}
endpoint.
const getTransactionStatus = async (vaultId, transactionId, accessToken) => {
const response = await fetch(`https://api.iofinnet.com/v1/vaults/${vaultId}/transactions/${transactionId}`, {
method: 'GET',
headers: {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json'
}
});
const resJson = await response.json();
return resJson.data;
};
Updated 27 days ago